Introduction
Choosing the ideal technology to use in developing websites and application is becoming more challenging in today’s digital landscape. This is as a result of the numerous tools and technology available on the digital market. However, React is gaining more momentum as more companies and businesses are utilizing it to create user interfaces. Released by Facebook in 2013, React or React.js is a popular, component based, open-source front end JavaScript library that is used to create intuitive websites and applications or user interfaces. This has led to the rising need of developers with proficiency in React. The rising demand of programmers or web developers proficient with React does not equal immediate employment. Like any other field, potential candidates also have to go through the employment process and acing an interview is one part. The interviews can be a bit overwhelming and that is why the need for preparation is important as it helps you be confident and ready to face any query or assessment thrown your way. In view of this, this article has put together 20 of top react interview questions to help equip developers with the relevant knowledge and insights to ace their interviews.
Basic Questions
What is React and why is it popular?
This question is usually serves as the curtain raiser to most react interview questions. React, simply put is an open-source JavaScript library used for building user interfaces. It is a framework that allows developers to build intuitive user interfaces especially for single page applications. Reacts efficiency in handling complex UI components contributes to its popularity.
Explain the virtual DOM
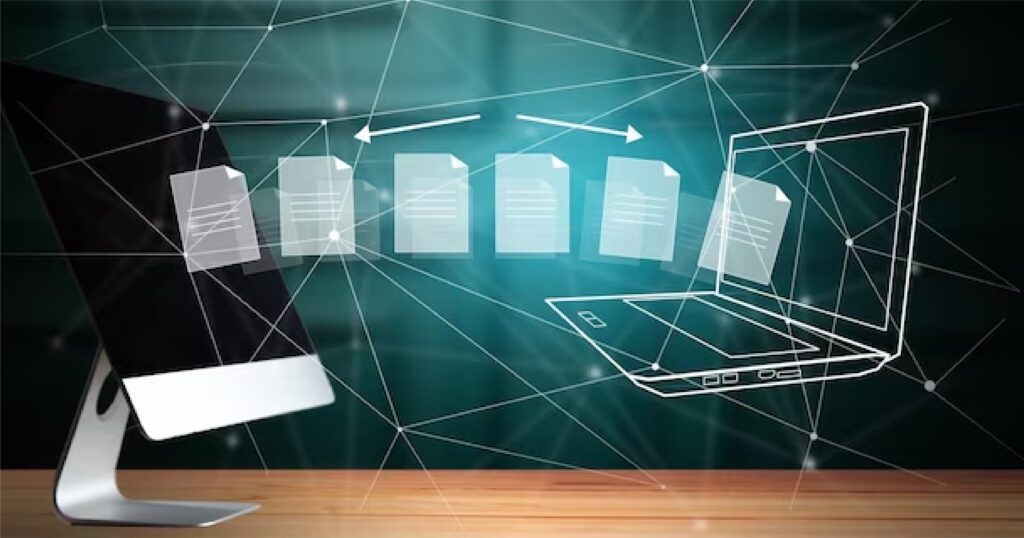
Next on our React interview questions list is virtual DOM. Document object model or DOM is a light weight representation of the actual DOM. Instead of updating the actual DOM React only updates the virtual DOM and that optimizes performance, ensuring that UI is responsive and and faster. This makes it possible for React to compare the updated and previous states quickly, figuring out what changes are really necessary and only updating those particular areas of the DOM.
Describe JSX and its importance.

The next item on our React interview questions list. JavaScript XML, or JSX, is an HTML- or XML-like syntax extension for JavaScript. It makes it easier for developers to create components with a familiar HTML structure by enabling them to write HTML-like code directly in JavaScript files. Because it makes it possible for developers to smoothly integrate JavaScript logic with the presentation layer, JSX is crucial for improving the readability and maintainability of code. JSX is converted to plain JavaScript so that browsers can understand it using Babel, a well-known JavaScript compiler.
Answers & Explanations
Benefits of React's component-based architecture.
React's component-based architecture enhances code reusability, maintainability, and scalability by encapsulating specific functionalities. This modularity allows developers to create complex applications by composing smaller, manageable pieces. Components can be reused across different parts or projects, saving time and effort. This approach also enhances collaboration among developers and facilitates testing by isolating individual components for unit testing, ensuring application reliability and stability.
React interview questions.
Advantages of the virtual DOM in performance.
The speed of React apps is greatly enhanced by the virtual DOM. React reduces the number of updates required by making a thin copy of the original DOM. React only updates the particular components that have changed, saving rendering times and improving the user experience by not re-rendering the entire DOM tree. This efficiency is especially important because it lowers the rendering's computational overhead for dynamic applications that receive frequent updates. Furthermore, the virtual DOM aids in resource optimization, enhancing the responsiveness and scalability of React applications.
How JSX simplifies UI development.
By offering a more user-friendly syntax and enabling developers to write HTML-like code inside JavaScript files, JSX streamlines the development of React user interfaces. It also makes sure that all browsers are compatible and assists in identifying syntax errors during development. By combining the strength of JavaScript with the familiarity of HTML, JSX improves the developer experience by making complex user interfaces (UIs) easier to visualize and construct. This makes it a powerful tool for creating dynamic, interactive interfaces.
Component Lifecycle & State Management
Describe React's component lifecycle methods.
React's component lifecycle consists of three phases: mounting, updating, and unmounting. The mounting phase initializes a component and inserts it into the DOM using methods like constructor(), static getDerivedStateFromProps(), render(), and componentDidMount(). The updating phase triggers changes in a component's state or props using methods like static getDerivedStateFromProps(), shouldComponentUpdate(), render(), getSnapshotBeforeUpdate(), and componentDidUpdate(). The unmounting phase removes the component from the DOM using componentWillUnmount().
How is state managed in React components?
Preparation of react interview questions and answers is not complete without this. In React, a component's state is its internal data. It can be initialized in the constructor() method and is managed by the this.state object. In order to cause a re-render and reflect the changes in the user interface, the state should be updated using the setState() method. React batches state updates for performance reasons because setState() is an asynchronous function. This implies that the updated state might not always be reflected right away. It's also advised to use the function setState() to guarantee accurate updates when changing a state that depends on a previous state.
What is the significance of hooks like `useState` and `useEffect`?
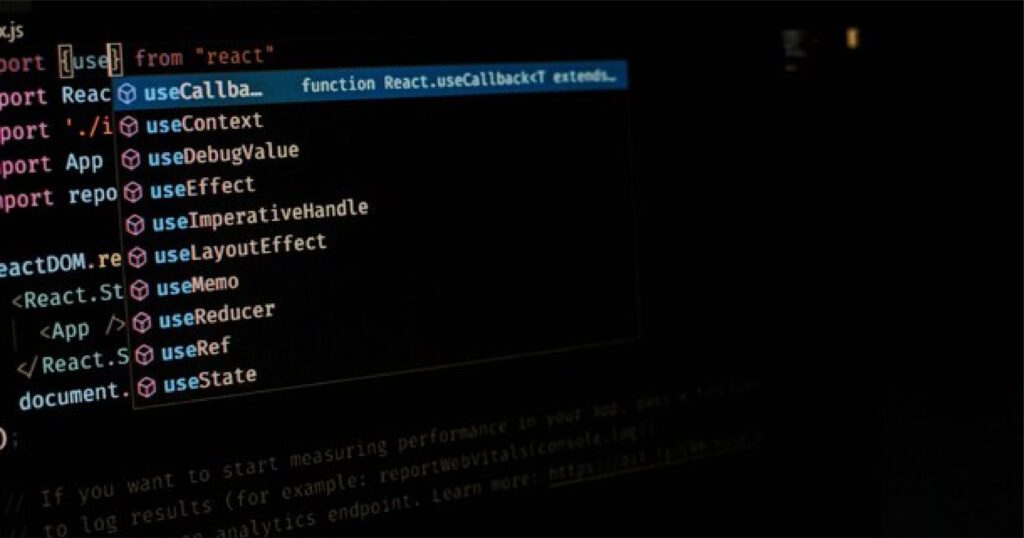
The next on our React interview questions list is this. Introduced in version 16.8, React's hooks allow functional components to control side effects and state. useState removes the requirement for class-based components by enabling local state management. useEffect replaces lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount in functional components to perform side effects. By doing away with the need for class components and promoting a more functional and declarative style of writing React code, these hooks simplify state management and side effects. They play a key role in contemporary React development.
Answers & Explanations
Lifecycle phases: Mounting, Updating, and Unmounting.
The mounting phase involves creating and inserting it into the DOM using constructor(), static getDerivedStateFromProps(), render(), and componentDidMount(). The updating phase triggers changes in the component's state or props, with static getDerivedStateFromProps(), shouldComponentUpdate(), render(), getSnapshotBeforeUpdate(), and componentDidUpdate() being called. The component is then removed from the DOM in the unmounting phase using componentWillUnmount(), allowing the developer to clean up resources like event listeners or timers.
State vs. Props in data handling.
A component's state is its internal data. This.setState() can be used to update it, and the this.state object is used to manage it. State is used for data that is unique to a particular component and is local to that component. Data is passed from a parent component to a child component using props, which is short for properties. They shouldn't be altered inside the child component since they are unchangeable. Props facilitate communication between parts, allowing smaller, reusable parts to be assembled into more complex applications.
Use cases for common hooks.
React interview questions can be derived from this statement.
By offering an array with the current state value and a function to update it, the useState hook adds state to functional components. It is employed to update and maintain internal state. Functional component lifecycle methods such as componentDidMount, componentDidUpdate, and componentWillUnmount are replaced by the useEffect hook, which handles side effects. Components can share common data without requiring props to be passed through the component tree by subscribing to context changes through the useContext hook. In complex applications, the useReducer hook is used for state management, while the useRef hook maintains values across renders. Especially for large datasets or complex computations, the useMemo and useCallback hooks optimize performance by memoizing pricey computations or functions.
Advanced Concepts & Practices
Advanced Questions
Explain context and how it differs from Redux.
This is one of react interview questions that cannot be treated lightly. When data needs to be accessed by multiple components at different levels of the tree, React's context feature makes it possible for data to be passed through the component tree without the need for manual props. It shares states with components that aren't linked directly by parent-child relationships. Redux is a JavaScript state management library that offers a global store for handling complicated state across several components. Context works well for specific use cases, such as localization or themes, while Redux is better at handling intricate global states in larger applications with interconnected parts.
What are React portals?
Another one of the ‘must prepare for’ react interview questions. A component can "portal" its content outside of its parent component by rendering its children into a different area of the DOM tree with the help of React Portals. When you need to render content in a different area of the DOM hierarchy, like a tooltip that must appear above all other elements or a modal, this is especially helpful.
ReactDOM.createPortal(child, container) is the method used to create portals; container is a reference to a DOM element where the content is to be rendered, and child is the component you want to render. By rendering content outside of the typical parent-child hierarchy, you can preserve component encapsulation and visually arrange elements on the page.
Describe the concept of Suspense and Concurrent Mode.
This is part of the likely react interview questions one must prepare for. Suspense in React allows components to suspend rendering while waiting for data to load, enhancing user experiences and handling asynchronous operations. It is often used in combination with React.lazy() and React's new concurrent rendering model. Concurrent Mode, also known as "Concurrent React," breaks down complex work into smaller units, ensuring the UI remains interactive even during expensive tasks. When combined, Suspense and Concurrent Mode significantly improve React applications' performance and user experience, enabling them to handle complex operations without freezing or becoming unresponsive.
Answers & Explanations
Context API vs. Redux in global state management.
Context API is a component tree-based method for sharing data without prop drilling, suitable for smaller applications or those needing global state. It's part of React core, making it easier to use. Redux is a dedicated state management library for JavaScript applications, providing a global store for managing complex state across multiple components. It's ideal for larger applications with intricate data flow patterns. Choose Context for simpler state management needs within a component tree, while Redux is suitable for complex, interconnected components or multipcurtin le parts needing access to the same state.
Use cases for portals in UI rendering.
Overlays and Modals: Tooltips, modal dialogs, and other UI elements that must appear above other content on the page can all be rendered using portals. This keeps encapsulation intact and guarantees that these elements are positioned correctly in the DOM hierarchy.
Render in a Different DOM Node: Portals give you the option to render content in a different DOM tree node, which is useful in situations where modals or other components need to exist outside of the typical parent-child relationship.
Z-index Management: Portals assist in controlling an element's z-index so that it appears above other elements on the page without affecting the parent component's style.
Performance benefits of Suspense and Concurrent Mode.
This can also be one of the many react interview questions to prepare for.
Suspense: Components can "suspend" rendering while they wait for data to load thanks to the suspense feature. This keeps the user interface from freezing and makes the experience more seamless, particularly for asynchronous tasks like data fetching. It lessens the requirement for intricate loading state control.
Concurrent Mode: By dividing complicated work into smaller, interruptible units, Concurrent Mode makes React more resilient and responsive. This guarantees that even during resource-intensive operations, the user interface stays interactive. When processing large data sets or rendering lengthy lists, it keeps the application from becoming sluggish or unresponsive.
Modern React Features & Updates in 2024
Trending Questions
How has React evolved since 2020?
This is also one of the many react interview questions you need to prepare for. Since 2020, React has made significant improvements, including the introduction of features like Concurrent Mode and Server Components, which enhance developer experience and simplify code. Concurrent Mode allows for multiple tasks to be worked on simultaneously, making applications more responsive. Server Components blurs the lines between server and client-rendered content, potentially revolutionizing rendering in React. React 18 has , which was recently released also improved rendering and concurrent rendering capabilities, making it more efficient and capable of handling complex UIs.
What are the latest features introduced in 2024?
Keeping current is a must in the digital landscape so expect more react interview questions like this. In 2024, React is still at the forefront of web development innovation. It is concentrating on Concurrent Mode, which enables React to work on several projects at once, improving responsiveness and user experience. Additionally, the React team is working on enhancing server-side rendering with Server Components, which could transform React rendering by blurring the lines between content rendered by server and client-rendered content. The 2024 updates demonstrate React's dedication to performance optimization. React 18 adds enhancements to rendering efficiency and concurrent rendering capabilities, guaranteeing that React-powered applications can manage intricate user interfaces with ease.
How is React improving web performance in this year's updates?
React interview questions like this one is no surprising during an interview. React's 2024 focus on web performance includes improvements in Concurrent Mode, which allows for multiple tasks to be performed simultaneously, reducing UI freezes and slowdowns. This feature enhances the responsiveness of React applications. The development of Server Components, still in its experimental phase, has the potential to improve performance by redefining rendering approaches. React's dedication to refining core features and introducing innovative concepts demonstrates its commitment to enabling developers to create high-performance web applications.
Closing Tips for React Interviews in 2024
To solidify your React skills, create hands-on projects that apply theoretical knowledge in practical scenarios. Stay updated with React's official documentation, which provides detailed explanations, examples, and best practices for using React effectively. Emphasize real-world problem-solving skills in interviews, highlighting instances of optimizing performance, implementing complex features, or resolving bugs while trying your hands on our top picked react interview questions. This will set you apart as a candidate who can apply React to real-world problems. React Interview questions helps highlight your ability to work effectively in a team, communicate clearly, and adapt to new technologies.
Conclusion
In conclusion, it is critical for any developer, novice or experienced, to stay up-to-date with React's dynamic evolution. Every day, the React ecosystem rolls out new game-changing features and methods. Adopt a mindset that values lifelong learning, experimentation with novel ideas, and technological proficiency. This guarantees that you stay at the forefront of web development in the constantly changing React landscape. Practicing and preparing with react interview questions affords you the confidence and opportunity to ace any interview and land your dream job.